Discover the easy steps to integrate the Razorpay payment gateway in your ReactJS app and start accepting payments effortlessly!
Introduction to Online Payments and Razorpay
Online payment solutions are a way for people to pay for things on the internet without using cash or checks. It’s like having a digital wallet that lets you buy stuff online. Ecommerce payment processing is the behind-the-scenes magic that makes it all work smoothly.
Explore hassle-free payments with Razorpay in ReactJS!
Stay updated with our Whatsapp newsletter for more tips and tutorials.
One cool tool for handling online payments is Razorpay. It’s like a virtual cash register that helps businesses get paid online. With Razorpay, you can securely and easily accept customer payments on your website.
Razorpay integration in react js and Node js
1. Create a Razorpay Account
Navigate to the Razorpay website and create an account. You can work in Test mode initially, so there’s no need to activate it.
After creating an account, go to the Settings tab in the Razorpay Dashboard and generate your API keys. Save these keys for later use.
2. Set Up Your Server
Create a folder on your computer and initialize npm in that folder using npm init
.
Install necessary dependencies by running:
npm install express razorpay dotenv crypto mongoose
JavaScriptCreate a server.js
file in the root directory and set up your Express server.
3. Save API Keys
Create a .env
file in the root directory.
Add your Razorpay API keys to the .env
file:
RAZORPAY_SECRET=<your razorpay secret>
RAZORPAY_KEY_ID=<your razorpay key id>
JavaScript4. Add Routes for Payment Handling
Create a new folder called routes
in the root directory.
Inside the routes
folder, create a file named payment.js
.
Set up a POST route in payment.js
to create an order using Razorpay API.
app.post("/order", async (req, res) => {
try {
const razorpay = new Razorpay({
key_id: process.env.RAZORPAY_KEY_ID,
key_secret: process.env.RAZORPAY_KEY_SECRET
});
if(!req.body){
return res.status(400).send("Bad Request");
}
const options = req.body;
const order = await razorpay.orders.create(options);
if(!order){
return res.status(400).send("Bad Request");
}
res.json(order);
} catch (error) {
console.log(error);
res.status(500).send(error);
}
})
JavaScript5. Create a React App
Generate a new React app inside the root directory using:
npm create vite@latest
JavaScriptInstall Axios for making requests to the backend by navigating to the client
folder and running:
npm install axios
JavaScript6. Add a Button to Initiate Payment Flow
In the App.js
file of the React app, replace the existing code with code that includes a button to initiate the payment flow.
import { useState } from 'react'
import './App.css'
function App() {
const paymentHandler = async (event) => {
const amount = 500;
const currency = 'INR';
const receiptId = '1234567890';
const response = await fetch('http://localhost:5000/order', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
amount,
currency,
receipt: receiptId
})
})
const order = await response.json();
console.log('order', order);
var option = {
key:"",
amount,
currency,
name:"Web Codder",
description: "Test Transaction",
image:"https://i.ibb.co/5Y3m33n/test.png",
order_id:order.id,
handler: async function(response) {
const body = {...response,}
const validateResponse = await fetch('http://localhost:5000/validate', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(body)
})
const jsonResponse = await validateResponse.json();
console.log('jsonResponse', jsonResponse);
},
prefill: {
name: "Web Coder",
email: "[email protected]",
contact: "9000000000",
},
notes: {
address: "Razorpay Corporate Office",
},
theme: {
color: "#3399cc",
},
}
var rzp1 = new Razorpay(option);
rzp1.on("payment.failed", function(response) {
alert(response.error.code);
alert(response.error.description);
alert(response.error.source);
alert(response.error.step);
alert(response.error.reason);
alert(response.error.metadata.order_id);
alert(response.error.metadata.payment_id);
})
rzp1.open();
event.preventDefault();
}
return (
<>
<div className='product'>
<h1>Razporpay Payment Getway</h1>
<button className='button' onClick={paymentHandler}>Pay Now</button>
</div>
</>
)
}
export default App
JavaScript7. Load Razorpay Checkout Script and Make a POST Request
Write a function App.js
to load the Razorpay checkout script and make a POST request to the backend to create a new order.
// Load this file in Index.html
<script src="https://checkout.razorpay.com/v1/checkout.js"></script>
JavaScriptUse the response from the backend to open the Razorpay popup for payment.
8. Verify Payment on the Server
Create a new POST route in payment.js
to handle successful payment verification.
Verify the payment using the details sent from the front end and the Razorpay API in this route.
app.post("/validate", async (req, res) => {
const {razorpay_order_id, razorpay_payment_id, razorpay_signature} = req.body
const sha = crypto.createHmac("sha256", process.env.RAZORPAY_KEY_SECRET);
// order_id + " | " + razorpay_payment_id
sha.update(`${razorpay_order_id}|${razorpay_payment_id}`);
const digest = sha.digest("hex");
if (digest!== razorpay_signature) {
return res.status(400).json({msg: " Transaction is not legit!"});
}
res.json({msg: " Transaction is legit!", orderId: razorpay_order_id,paymentId: razorpay_payment_id});
})
JavaScript9. Test Payment Flow
Use the provided demo card details to test the payment flow in Test mode.
Once you’ve tested successfully, you can switch to Live mode and activate your account for production.
10. Final Steps
You can find all the source codes here.
Consider writing a blog post about integrating Stripe with React in the future.
By following these steps, you can integrate the Razorpay payment gateway into your React application successfully.
What is Razorpay?
Have you ever wondered how to pay for things online without using cash? Well, that’s where Razorpay comes in! Razorpay is like a unique tool that helps people pay for things on the internet safely and easily. It’s like having your digital wallet that you can use to buy stuff without handling physical money.
Imagine you want to buy a new video game online. Instead of going to a store and giving cash to the cashier, you can use Razorpay to pay for the game right from your computer or phone. It’s super convenient and saves you the hassle of dealing with paper money. Plus, it’s really secure, so you don’t have to worry about your payment information getting into the wrong hands.
Learn how to easily setup Razorpay in ReactJS and start accepting payments with just a few lines of code! Click Here #webdevelopment #paymentsolution
Web Codder
Why Use Razorpay in Your ReactJS Project?
In this part, we’ll explain why ReactJS is a smart choice for making websites and how adding Razorpay to your ReactJS project can make getting money from customers super smooth.
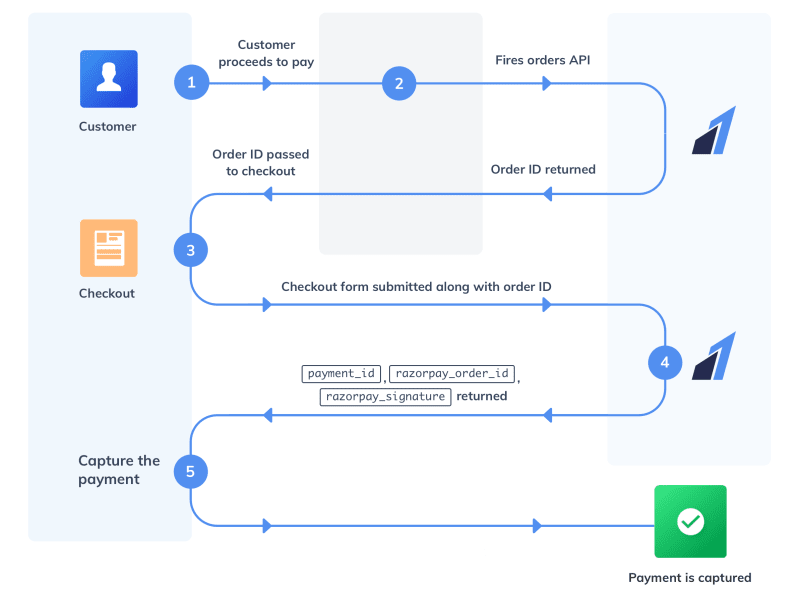
ReactJS is a cool tool that helps developers create awesome websites. It makes things look great and work smoothly. When you add Razorpay to your ReactJS project, it becomes easy for people to pay for things on your website. Razorpay is like a digital cashier that helps handle the money stuff securely and quickly.
Imagine you have a lemonade stand online. With ReactJS and Razorpay, your customers can easily pay for their lemonade with just a few clicks. It’s like having a magic button that turns their money into your lemonade!
By using Razorpay in your ReactJS project, you can make buying and selling things online a breeze. Customers will love how simple it is to pay, and you’ll love how easy it is to get paid.
Setting Up Razorpay in Your ReactJS Project
Now, we’ll show you step-by-step how to put Razorpay into your ReactJS website so you can start getting paid!
Create a Razorpay Account
First things first, you need to sign up for Razorpay before you can use it.
Install Razorpay’s ReactJS Package
We’ll guide you through how to add the special Razorpay code to your ReactJS project.
Configure Your Payment Gateway
Here, you’ll learn how to tell Razorpay about your website so it can handle the money stuff.
Testing Your Payment Gateway
Before you start using it for real, we’ll show you how to test Razorpay to make sure everything works perfectly.
Tips for a Successful Razorpay Integration
Integrating Razorpay into your ReactJS project can be a game-changer for your online business. To ensure a smooth and successful integration, here are some tips to keep in mind:
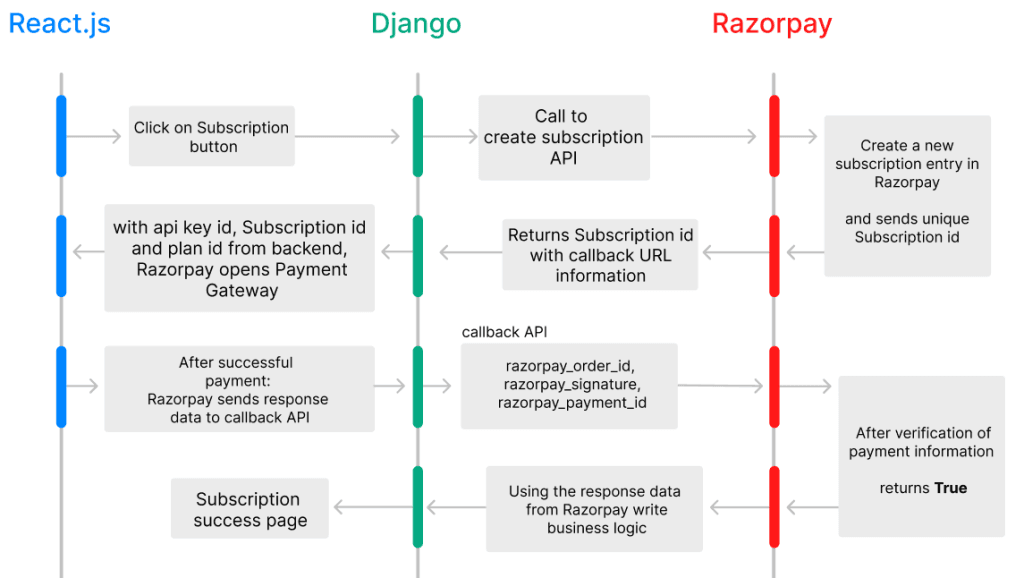
Customize Your Razorpay Integration
When setting up Razorpay in your ReactJS project, take advantage of the customization options available. Tailor the payment gateway to match your website’s branding and design for a seamless user experience.
Stay Updated with Razorpay Documentation
Razorpay offers comprehensive documentation and guides to help you navigate the integration process. Stay updated with their latest resources to make the most of the payment gateway’s features and functionalities.
Test Thoroughly Before Going Live
Prior to launching your website with Razorpay integration, conduct thorough testing to ensure that all payment processes function correctly. Test different scenarios, such as successful payments, failed transactions, and refunds, to guarantee a hassle-free experience for your customers.
Provide Clear Instructions for Users
Make sure to provide clear instructions for users on how to complete payments using Razorpay on your website. A simple and intuitive checkout process can help reduce cart abandonment rates and improve overall user satisfaction.
By following these tips, you can set up a successful Razorpay integration in your ReactJS project and streamline the online payment process for your customers.
Conclusion
In conclusion, setting up Razorpay with ReactJS can revolutionize the way you handle online payments for your business. By integrating this payment gateway, you can provide your customers with a seamless and secure transaction experience, ultimately leading to increased trust and satisfaction.
With Razorpay, you have access to a reliable online payment solution that simplifies the process of accepting payments on your website. This not only enhances the user experience but also streamlines your ecommerce payment processing, making it easier for you to manage your online transactions efficiently.
By following the steps outlined in this guide, you can configure Razorpay in your ReactJS project with ease. From creating a Razorpay account to testing your payment gateway, each step is designed to help you seamlessly integrate this payment solution into your website.
Overall, Razorpay’s ReactJS integration offers a convenient and secure way to handle online payments, making it a valuable addition to any ecommerce platform. So, why wait? Start using Razorpay today and take your online shop to the next level!